Try something like this in a PythonCaller (assuming Python 3):
import fmeobjects
import csv
class multi_string_replace(object):
def __init__(self):
with open(r'C:\my_streets\street_mapping.csv') as csvfile:
self.street_mappings = dict(csv.reader(csvfile, delimiter=';'))
def input(self, feature):
street_name = feature.getAttribute('street_name_attribute')
if street_name:
for search_name, replace_name in self.street_mappings.items():
street_name = street_name.replace(search_name, replace_name)
feature.setAttribute('street_name_attribute', street_name)
self.pyoutput(feature)
This will read the file 'C:\my_streets\street_mapping.csv' once at startup, caching all the search-replace pairs in a Python dictionary. For each feature that enters the PythonCaller, the attribute street_name_attribute will be processed to replace all the names found in the street name attribute with the corresponding values from the csv.
Try something like this in a PythonCaller (assuming Python 3):
import fmeobjects
import csv
class multi_string_replace(object):
def __init__(self):
with open(r'C:\my_streets\street_mapping.csv') as csvfile:
self.street_mappings = dict(csv.reader(csvfile, delimiter=';'))
def input(self, feature):
street_name = feature.getAttribute('street_name_attribute')
if street_name:
for search_name, replace_name in self.street_mappings.items():
street_name = street_name.replace(search_name, replace_name)
feature.setAttribute('street_name_attribute', street_name)
self.pyoutput(feature)
This will read the file 'C:\my_streets\street_mapping.csv' once at startup, caching all the search-replace pairs in a Python dictionary. For each feature that enters the PythonCaller, the attribute street_name_attribute will be processed to replace all the names found in the street name attribute with the corresponding values from the csv.
Thank you @david_r! I am using Python 3.7.
I changed csv-filename and attribute name, but I get error and I don't know why
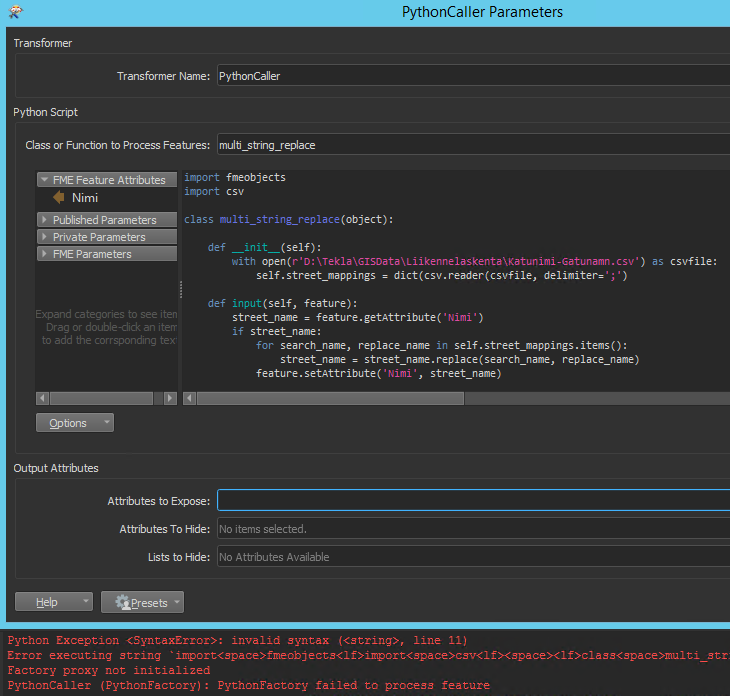
Thank you @david_r! I am using Python 3.7.
I changed csv-filename and attribute name, but I get error and I don't know why
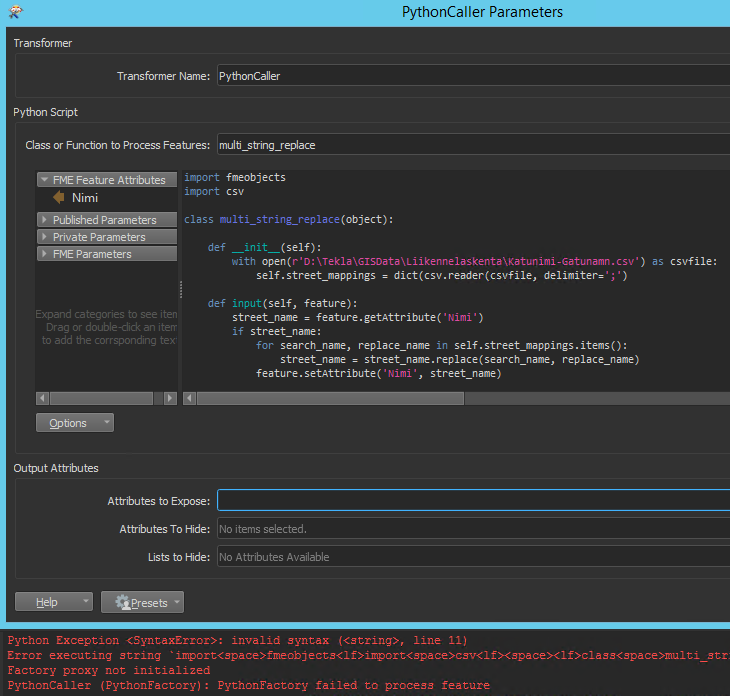
The code above was untested and contained two errors, I've fixed them now. Hopefully it works as promised.
Thank you @david_r! I am using Python 3.7.
I changed csv-filename and attribute name, but I get error and I don't know why
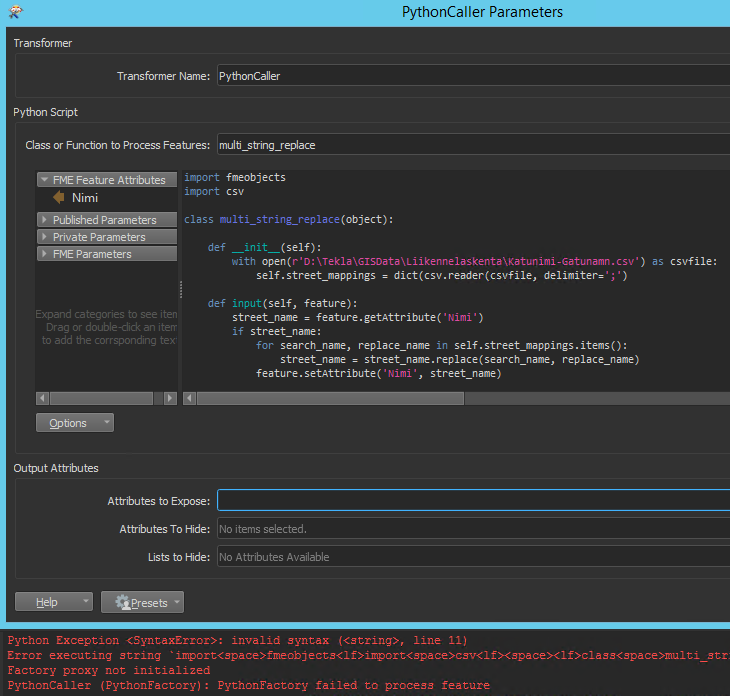
hi @bazooka, i had this error before with using wrong indentations... Everything looked nice, but i used a mix of spaces and tabs: not OK.... Under options you can enable 'Show spaces/tabs' (and line numbers too by the way).
Maybe worth checking this out first?
hi @bazooka, i had this error before with using wrong indentations... Everything looked nice, but i used a mix of spaces and tabs: not OK.... Under options you can enable 'Show spaces/tabs' (and line numbers too by the way).
Maybe worth checking this out first?
I had forgotten a closing parenthesis on line 8, unfortunately... Fixed now.
But yes indeed, indentations are a common issue when copy/pasting code, in particular from forums like these.
I had forgotten a closing parenthesis on line 8, unfortunately... Fixed now.
But yes indeed, indentations are a common issue when copy/pasting code, in particular from forums like these.
Now code works! Thanks again!