I am using a python Caller to determine if a value is in a range of two other values which are read from a csv file.
For Example: Is SearchValue LMH_HNR_Z in the range of value PTOa and value PTOb? If yes, set the variable Fall = B3, if not set it to B3f.
LMH_HNR_Z = 5, PTOa = 1, PTOb = 10 should return: Fall=3B,
LMH_HNR_Z = 11, PTOa=1, PTOb=10 should return: Fall=3Bf
The Code in this picture is part of a bigger function where I discovered the problem but I narrowed the problematic part down to this. The row where the problem happens is this:
if (min(PTOa, PTOb) <= LMH_HNR_Z <= max(PTOa, PTOb)):
What happens is, that this request randomly returns false even if the search value LMH_HNR_Z is in the range of PTOa and PTOb. The output of some tested values and ranges is also visible in the lower part of this post. I can’t really see a pattern there, maybe one of you?
Is someone aware of a bug that causes this behavior? I am using FME Data Interoperability, FME(R) 2022.2.1.0 (20221202 - Build 22776 - WIN64)
Thanks in advance.
Here is the Code I#m using in the PythonCaller:
import fme
import fmeobjects
import re
# Template Class Interface:
# When using this class, make sure its name is set as the value of
# the 'Class or Function to Process Features' transformer parameter
class FeatureProcessor(object):
def __init__(self):
pass
def input(self,feature):
print('Starte Python-Falluntersuchung für Bereich 1')
LMH_HNR_Z = feature.getAttribute('LMH_HNR_Nr')
#PTO = feature.getAttribute('PTO_schriftinhalt')
PTOa = feature.getAttribute('PTO_schriftinhalt_A')
PTOb = feature.getAttribute('PTO_schriftinhalt_B')
print('PTOa: ', PTOa)
print('PTOb: ', PTOb)
print('LMH_HNR_Z:',LMH_HNR_Z)
if (min(PTOa, PTOb) <= LMH_HNR_Z <= max(PTOa, PTOb)):
print('Minab: ',min(PTOa, PTOb))
print('Maxab: ',max(PTOa, PTOb))
print('LMH_HNR_Z_ok:',LMH_HNR_Z)
feature.setAttribute("Fall",'B3')
print('FOUND B3')
else:
feature.setAttribute("Fall",'B3f')
print('Minab: ',min(PTOa, PTOb))
print('Maxab: ',max(PTOa, PTOb))
print('LMH_HNR_Z_fehler:',LMH_HNR_Z)
print('FOUND B3f')
self.pyoutput(feature)
def close(self):
pass
Example Output:
LMH_hausnummer; PTO_schriftinhalt; PTO_schriftinhalt_A; PTO_schriftinhalt_B
2 1-10 2 B3f 1 10
2 1-11 2 B3f 1 11
2 1-12 2 B3f 1 12
2 1-13 2 B3f 1 13
2 1-14 2 B3f 1 14
2 1-15 2 B3f 1 15
2 1-16 2 B3f 1 16
2 1-17 2 B3f 1 17
2 1-18 2 B3f 1 18
2 1-19 2 B3f 1 19
2 1-20 2 B3 1 20
3 1-20 3 B3f 1 20
4 1-20 4 B3f 1 20
5 1-20 5 B3f 1 20
6 1-20 6 B3f 1 20
7 1-20 7 B3f 1 20
8 1-20 8 B3f 1 20
9 1-20 9 B3f 1 20
10 1-20 10 B3 1 20
11 1-20 11 B3 1 20
12 1-20 12 B3 1 20
13 1-20 13 B3 1 20
19 10-20 19 B3 10 20
19 11-20 19 B3 11 20
19 12-20 19 B3 12 20
19 13-20 19 B3 13 20
19 14-20 19 B3 14 20
19 15-20 19 B3 15 20
19 16-20 19 B3 16 20
19 17-20 19 B3 17 20
19 18-20 19 B3 18 20
11 10-20 11 B3 10 20
12 11-20 12 B3 11 20
13 12-20 13 B3 12 20
14 13-20 14 B3 13 20
15 14-20 15 B3 14 20
16 15-20 16 B3 15 20
17 16-20 17 B3 16 20
18 17-20 18 B3 17 20
19 18-20 19 B3 18 20
5 7-2 5 B3 7 2
6 1-12 6 B3f 1 12
3 1-5 3 B3 1 5
7 1-10 7 B3f 1 10
8 1-11 8 B3f 1 11
9 1-12 9 B3f 1 12
2 1-5 2 B3 1 5
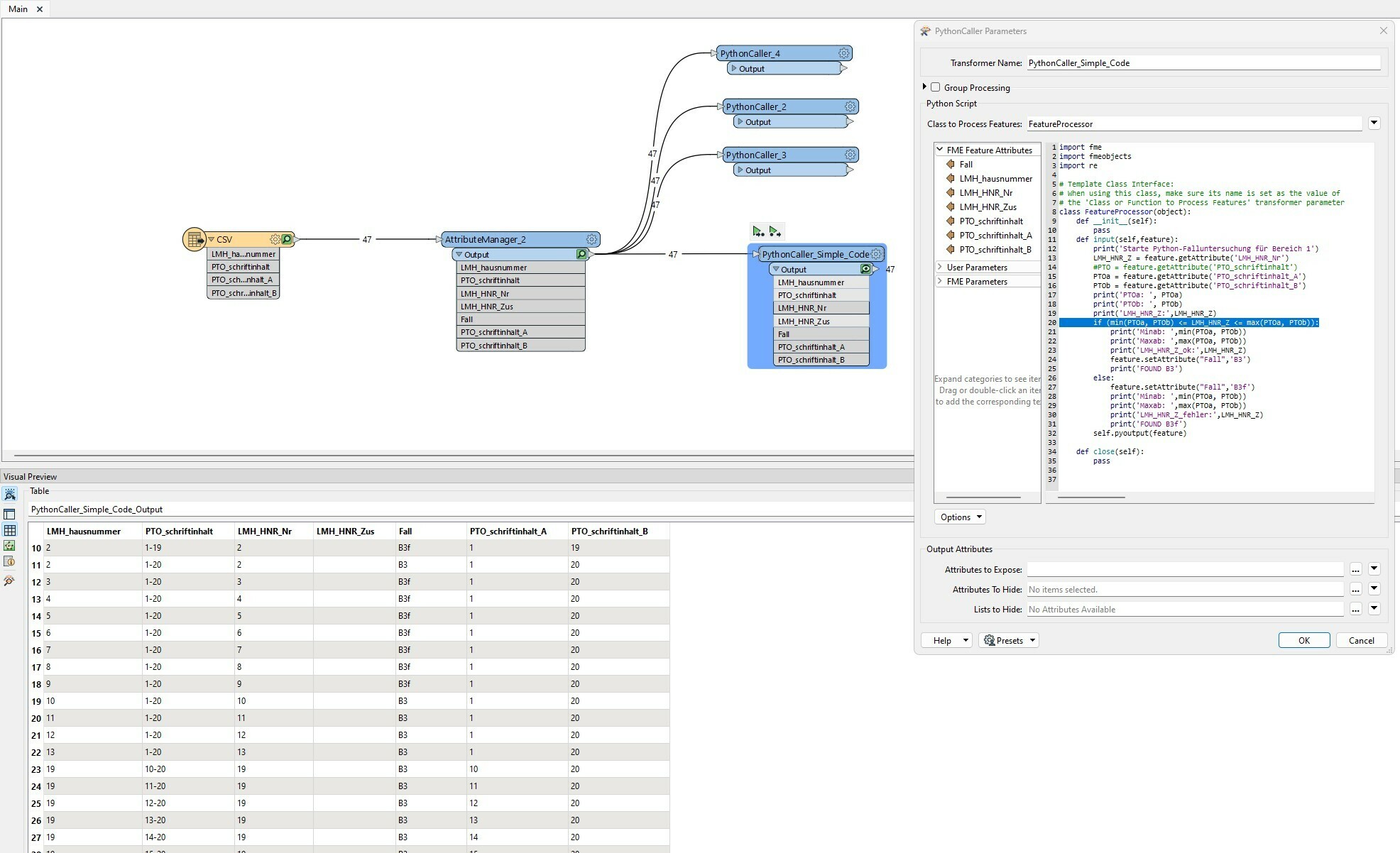