Here is a quick solution to remove the nth character from a string. I know there is probably a better way to do it in regex, but here is one way. See the attached workspace. Just select the attribute you wish to modify and type the index of the character (start at 1 instead of 0).
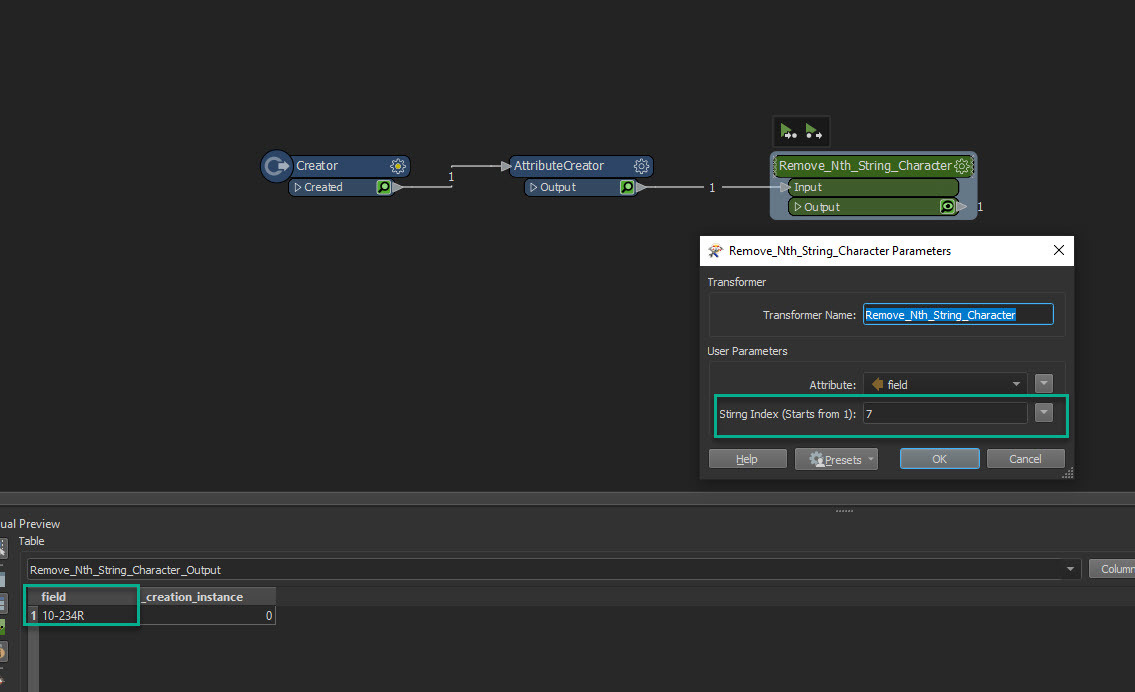
You can use either StringReplacer with a RegEx pattern, or AttributeCreator using the standard Left() and Substring() functions to achieve the same effect (and the formula using AttributeCreator is bascially the same as you would achieve it with Excel Left() and Mid() functions)
StringReplacer Solution
- Set (Group 1) as the first (n-1) characters: For example n=3, this is (^.{2}).(.*)
- Set (Group 2) as the characters after the nth character: this is (^.{2}).(.*)
- Return the result Group 1+Group 2 (ie. All Characters except the nth character)
So, set:
Text To Replace:
(^.{@sub(@Value(n),1)}).(.*)
Replacement Text:
\1\2
ie. This is a RegEx replacement string expression of Group 1+Group 2
Note, will need a Tester in front of this to check that there are at least n characters in the string, if not, leave the string value unprocessed ie. Don't send the Feature to StringReplacer.
The expression to test in the Tester is either the less typing way, or the fastest to calculate way:
- If Feature Value Contains RegEx RegExExpression Above; or alternatively use the probably faster to calculate......
- If @StringLength(@Value(StringAttribute)) >= @Value(n)
AttributeCreator Solution
Using the same method, but just with the different syntax for an AttributeCreator FME expression
- Set Group 1 to Left(StringToProcess,(n-1))
- Set Group 2 to Substring(StringToProcess,(n+1))
- Return result Group 1 + Group 2
Expression is:
@Left(@Value(StringAttribute),@sub(@Value(n),1))@Substring(@Value(StringAttribute),@add(@Value(n),1))
This will need to be used with Conditional Values in AttributeCreator:
- If String Length > n characters, then use the formula above
- Else If String Length exactly n characters, then use the formula above, but without the @Substring() component and only with the @Left component group
- Else Return the String Value unchanged
Hi, I know there are already some good answers here, but I also want to give mine. My solution use 2 SubstringExtractor transformers and 1 StringConcatenator to give you the solution.
I attached the workspace. The index of the char that you need to remove can be change with the published parameter in this workspace.
Thanks