In the past I've done this with a TCLCaller, basically telling FME to execute the @Value() function. For your _description attribute:
FME_Execute eFME_GetAttribute _description]
You'll also have to SubstringExtractor the result from 1 to -3 but it should work.
Also the TCLCaller is on the list to be deprecated for 2024. I'd be interested to know how to do this in Python.
If it is a finite amount of attributes (such as the 6 you posted here), I would opt for a StringReplacer, set it to Replace Regular Expression in the _description attribute and replace Regex "\\@Value\\(Name\\)" with "@Value(Name)".
Hi @lak you mention that "The values that are referenced in the Feature Description column are not exposed, but I can view the values when I click on a feature in the visual preview." However, having these attributes exposed is a requirement for the @Value() function to pull from those attributes. You can expose these easily using an AttributeExposer. Let us know if after they are exposed if you have any luck with your workflow.
@joepk and @Evie Lapalme, unfortunately the amount of attributes are not known and are definitely greater than the 6 I posted - the screenshot of the spreadsheet above was a dummy/testing one, the real one would be a couple hundred rows, all with different combination of different values strung together for the Feature Description.
AttributeExposer would do the trick but I couldn't see a way to dynamically expose attributes, and could only see ways to expose attributes manually by typing them in, and only if the attribute name is already known. Ideally I would like AttributeExposer to be able to dynamically expose by accepting an @Value() input.
@ctredinnick , TCLCaller worked an absolute treat - it did exactly what I expected the output to be if AttributeCreator could somehow expose the values. However, as you mentioned it being deprecated soon, I've been looking at other more future-proof methods.
I managed to solve the issue by using PythonCaller to replicate the functionality of TCLCaller, and I modified my spreadsheet slightly just for ease of input:
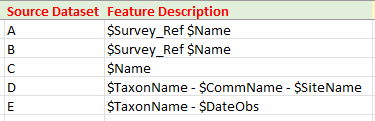
And in the PythonCaller:
import fme
import fmeobjects
import re
def makeDescription(feature):
#featureDesciption_Input = '$First - Test'
featureDesciption_Input = feature.getAttribute('Feature Description')
# split the description into a list of words and delimiters
fdSplit = re.split('(\W)', featureDesciption_Input)
previousValue = ""
featureDesciption_Output = ""
#loop through and replace the placeholder fields with the values
for f in fdSplit:
word = f
# if previous value is $, then this value will be the variable name.
if previousValue == "$":
word = feature.getAttribute(f)
if word != "$":
featureDesciption_Output = featureDesciption_Output + word
previousValue = f
# remove empty spaces and dashes where no value - order is important
featureDesciption_Output = featureDesciption_Output.replace(" - - "," - ")
# if first value is empty
featureDesciption_Output = featureDesciption_Output.replace(" - ","")
# if last value is empty
featureDesciption_Output = featureDesciption_Output.replace(" - ","")
feature.setAttribute('_description', featureDesciption_Output)
And I get the intended output in _description:
Thanks everyone for the suggestions and ideas.