this is the error
Python Exception <ValueError>: invalid literal for int() with base 10: ''
Error encountered while calling function `processFeature2'
PythonFactory failed to process feature
A fatal error has occurred. Check the logfile above for details
Bridge failed to output feature on tag `PYOUTPUT'
PythonFactory failed to process feature
Hi
Looks like Start_Mileage can sometimes be an empty string, you will have to handle that to avoid or ignore the ValueError exception. Something like:
vStartMiles = feature.getAttribute('Start_Mileage')
try:
vStartMiles2 = int(vStartMiles)
feature.setAttribute("Start_Miles", vStartMiles2)
except:
pass
Notice the try/except-block that ignores any potential errors when casting vStartMiles into an integer. You could easily adapt the above to treat these special cases as you see fit.
Now, having said that, I'm not quite sure what you're trying to accomplish with the above. FME is normally pretty good at casting its attributes to integers when necessary, without user intervention.
David
Thanks David. I've filtered out the features with empty strings before the Python caller and it works ok. However, the int function doesn't seem to work on a decimal string but vStartMiles2 = int(float(vStartMiles)) does
Eric
Thanks David. I've filtered out the features with empty strings before the Python caller and it works ok. However, the int function doesn't seem to work on a decimal string but vStartMiles2 = int(float(vStartMiles)) does
Eric
That is correct, you cannot cast a string looking like a float as an integer.
Be aware, however, that int(2.9) = 2 and not 3! Use round(float(vStartMiles) to get the value 3.
David
Hi ericarmitage,
As david_r already mentioned, it is important to use the round function when converting from a float towards an integer. Below I also implemented a try-except block to show a warning in the log if the conversion fails.
import fme
import fmeobjects
logger = fmeobjects.FMELogFile()
# Template Function interface:
def convertToInteger(feature):
string = feature.getAttribute('string')
try:
integer = float(string)
integer = round(integer)
integer = int(integer)
feature.setAttribute('integer', integer)
except:
logger.logMessageString('The following string couldn\'t be converted towards an integer: %s.' % (string), fmeobjects.FME_WARN)
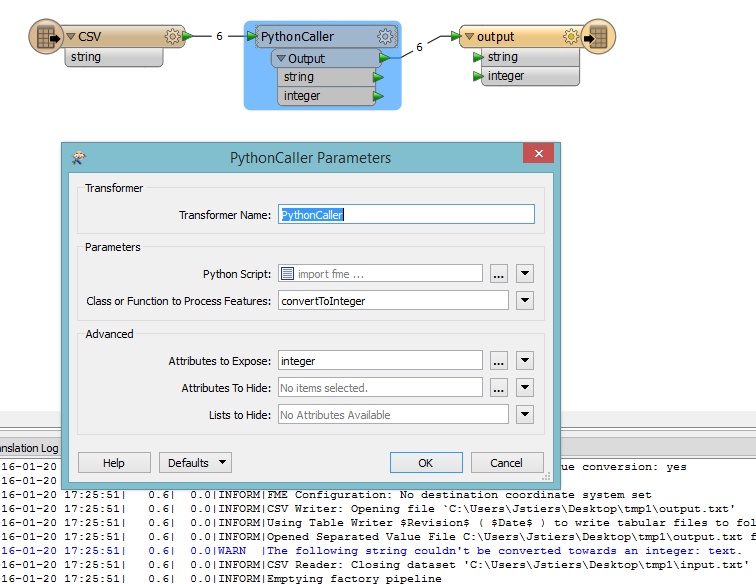
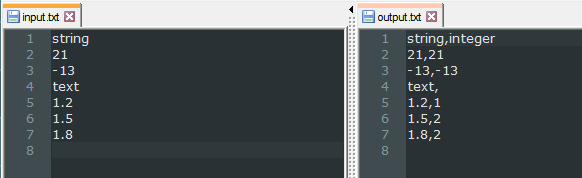