Hello Dries,
First of all, you can access FME factories documentation here: http://docs.safe.com/fme/html/FME_FactFunc/index.h...
And here is how I use a factory with python.
If I have this transformer in FME Workbench:
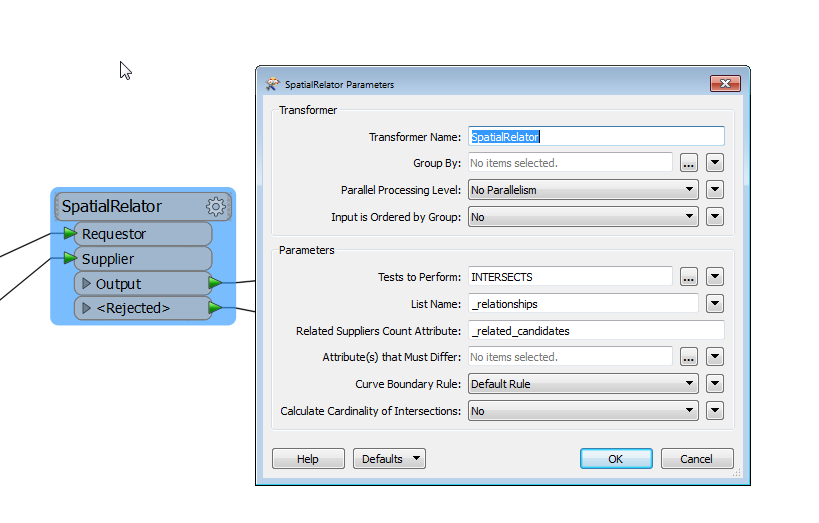
I can cut&paste; it in a text editor to see the factory code:
DEFAULT_MACRO WB_CURRENT_CONTEXT
FACTORY_DEF * SpatialRelationshipFactory \
FACTORY_NAME SpatialRelator \
INPUT BASE FEATURE_TYPE AttributeCreator_OUTPUT_0_olPiq+C1D00= \
INPUT CANDIDATE FEATURE_TYPE AttributeCreator_2_OUTPUT_1_KpUyI6tMDVI= \
PREDICATE "INTERSECTS" \
LIST_NAME "_relationships" \
SUCCESS_ATTR "_related_candidates" \
REJECT_INVALID_GEOM Yes \
CURVE_BOUNDARY_RULE ENDPOINTS_MOD2 \
CALCULATE_CARDINALITY No \
OUTPUT TAGGED FEATURE_TYPE SpatialRelator_Output \
OUTPUT REJECTED FEATURE_TYPE SpatialRelator_<Rejected>
I can then use that factory code in a python caller this way (note that I renamed some parameters and cleaned up some quotes (")):
import fme
import fmeobjects
class FeatureProcessor(object):
def __init__(self):
strFactory = """FACTORY_DEF * SpatialRelationshipFactory \
FACTORY_NAME SpatialRelator \
INPUT BASE FEATURE_TYPE RequestorPort \
INPUT CANDIDATE FEATURE_TYPE SupplierPort \
PREDICATE INTERSECTS \
LIST_NAME _relationships \
SUCCESS_ATTR _related_candidates \
REJECT_INVALID_GEOM Yes \
CURVE_BOUNDARY_RULE ENDPOINTS_MOD2 \
CALCULATE_CARDINALITY No \
OUTPUT TAGGED FEATURE_TYPE SpatialRelator_Output \
OUTPUT REJECTED FEATURE_TYPE SpatialRelator_<Rejected>"""
self.pipeline = fmeobjects.FMEFactoryPipeline("MyFactory")
self.pipeline.addFactory(strFactory)
def input(self,feature):
type = feature.getAttribute("_type")
if type == "LINE":
print "Adding a line"
feature.setFeatureType("RequestorPort")
self.pipeline.processFeature(feature)
elif type == "AREA":
print "Adding an area"
feature.setFeatureType("SupplierPort")
self.pipeline.processFeature(feature)
else:
raise Exception("Unexpected feature!")
def close(self):
myFeature = fmeobjects.FMEFeature()
myFeature.addCoordinates([(10,30),(100,30)])
myFeature.setFeatureType("RequestorPort")
self.pipeline.processFeature(myFeature)
self.pipeline.allDone()
tmpFeature = self.pipeline.getOutputFeature()
while tmpFeature != None:
if tmpFeature.getFeatureType() == "SpatialRelator_Output":
self.pyoutput(tmpFeature)
tmpFeature = self.pipeline.getOutputFeature()
<br>
Sample workspace is attached (FME 2015).
Regards,
Larry