I need some ideas on how to implement this workflow on my solution. After the HTML page is written a python caller will open the html page created. Sample code is appreciated. I'd like to use some format parameters of the HTML page created by FME, e.g. file path or directory so I could pass that to my python caller. What is the most efficient python library to use to open a URL? Is there a library that comes with ArcMap and FME install available?
If you need to access the page after it has been written, it might be that a shutdown script will work better than a PythonCaller, but the principle is the same.
These two modules should give you a good starting point:
For opening URL resources: urllib2 and tutorial
For parsing HTML, here's one way: HTMLParser
Tons of examples out there if you google a bit.
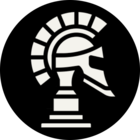
Thanks for the quick reply.
Before I read your suggestion I tried this using a networks share path to the html file:
import urllib
urllib.request.urlopen("//alxapfs23/RSALVALE$/2016/DGN_Conversions/Solution/output/Helloworld.htm").read()
And I got this error:
2016-07-21 07:20:09| 1.2| 0.1|ERROR |Python Exception <AttributeError>: 'module' object has no attribute 'request'
2016-07-21 07:20:09| 1.2| 0.0|ERROR |Traceback (most recent call last):
File "<string>", line 3, in <module>
AttributeError: 'module' object has no attribute 'request'
2016-07-21 07:20:09| 1.2| 0.0|ERROR |Error executing string `import urllib
urllib.request.urlopen("//alxapfs23/RSALVALE$/2016/DGN_Conversions/Solution/output/Helloworld.htm").read()'
2016-07-21 07:20:09| 1.2| 0.0|FATAL |Factory proxy not initialized
2016-07-21 07:20:09| 1.2| 0.0|FATAL |f_17(PythonFactory): PythonFactory failed to process feature
2016-07-21 07:20:09| 1.2| 0.0|ERROR |BADNEWS: A fatal error has occurred. Check the logfile above for details (dynafact.cpp:315)
2016-07-21 07:20:09| 1.2| 0.0|INFORM|=-=-=-=-=-=-=-=-=-=-=-=-=-=-=-=-=-=-=-=-=-=-=-=-=-=-=-=-=-=-=-=-=-=-=-=-=-=-=-
2016-07-21 07:20:09| 1.2| 0.0|INFORM|Feature output statistics for `FFS' writer using keyword `W_1':
2016-07-21 07:20:09| 1.2| 0.0|STATS |=-=-=-=-=-=-=-=-=-=-=-=-=-=-=-=-=-=-=-=-=-=-=-=-=-=-=-=-=-=-=-=-=-=-=-=-=-=-=-
2016-07-21 07:20:09| 1.2| 0.0|STATS | Features Written
2016-07-21 07:20:09| 1.2| 0.0|STATS |=-=-=-=-=-=-=-=-=-=-=-=-=-=-=-=-=-=-=-=-=-=-=-=-=-=-=-=-=-=-=-=-=-=-=-=-=-=-=-
2016-07-21 07:20:09| 1.2| 0.0|STATS |==============================================================================
2016-07-21 07:20:09| 1.2| 0.0|STATS |Total Features Written 0
2016-07-21 07:20:09| 1.2| 0.0|STATS |=-=-=-=-=-=-=-=-=-=-=-=-=-=-=-=-=-=-=-=-=-=-=-=-=-=-=-=-=-=-=-=-=-=-=-=-=-=-=-
2016-07-21 07:20:09| 1.2| 0.0|ERROR |A fatal error has occurred. Check the logfile above for details
2016-07-21 07:20:09| 1.2| 0.0|ERROR |(stftrans.cpp:293) - (simptran.cpp:769) - (stftrans.cpp:323) - (pipeline.cpp:992) - (fctwriter.cpp:673) - (fctwriter.cpp:697) - (factory.cpp:1183) - (..\..\foundation\framework\engine\stfspec.h:229) - (factory.cpp:1146) - (pipeline.cpp:1149) - (pipeline.cpp:760) - (..\..\foundation\framework\engine\stfspec.h:229) - (factory.cpp:539) - (fcttee.cpp:232) - (fcttee.cpp:259) - (..\..\foundation\framework\engine\stfspec.h:229) - (factory.cpp:1146) - (pipeline.cpp:1149) - (pipeline.cpp:760) - (..\..\foundation\framework\engine\stfspec.h:229) - (factory.cpp:539) - (fcttee.cpp:239) - (fcttee.cpp:259) - (..\..\foundation\framework\engine\stfspec.h:229) - (factory.cpp:1146) - (pipeline.cpp:1149) - (pipeline.cpp:765) - (factory.cpp:539) - (fctcreat.cpp:500) - (factory.cpp:1146) - (pipeline.cpp:1149) - (pipeline.cpp:760) - (..\..\foundation\framework\engine\stfspec.h:229) - (factory.cpp:539) - (dynafact.cpp:315)
2016-07-21 07:20:09| 1.2| 0.0|INFORM|FME Session Duration: 1.4 seconds. (CPU: 0.5s user, 0.4s system)
2016-07-21 07:20:09| 1.2| 0.0|INFORM|END - ProcessID: 12844, peak process memory usage: 72108 kB, current process memory usage: 72104 kB
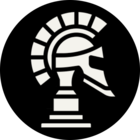
I fixed the fatal error by inserting the code in the def processFeature that comes by default with the PythonCaller.
The URL still did not open but the good thing is my translation ran without failure.
def processFeature(feature):
response = urllib2.urlopen('file:////alxapfs23/RSALVALE$/2016/DGN_Conversions/Solution/output/Helloworld.htm')
html = response.read()
pass
I fixed the fatal error by inserting the code in the def processFeature that comes by default with the PythonCaller.
The URL still did not open but the good thing is my translation ran without failure.
def processFeature(feature):
response = urllib2.urlopen('file:////alxapfs23/RSALVALE$/2016/DGN_Conversions/Solution/output/Helloworld.htm')
html = response.read()
pass
It depends on what you mean by "open" the URL. Your code does open the URL and reads the contents it into an object (variable) you create called "html" on line 3, but then you let FME continue withouth doing anything with neither the object or the contents.
Maybe you mean that you want to open the html page in your default browser? If so that's much easier, look e.g. here: https://pythonconquerstheuniverse.wordpress.com/2010/10/16/how-to-open-a-web-browser-from-python/
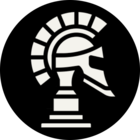
@david_r, I found that link too and almost gave up on it after frustratingly trying every possible combinations of network share path. I gave up on the PythonCaller and instead used the Shutdown script you suggested then used sample code on that link.
Here's my final code, which also includes the path that worked for me
import fme
import fmeobjects
import webbrowser
new = 2 # open in a new tab, if possible
# open a public URL, in this case, the webbrowser docs
# url = "http://docs.python.org/library/webbrowser.html"
# webbrowser.open(url,new=new)
# open an HTML file on my own (Windows) computer
url = "file://///H:/2016/DGN_Conversions/Solution/output/HelloWorld.html"
webbrowser.open(url,new=new)
Thanks a lot... now I have to learn HTMLReportGenerator and then test the File and Path transformer to parameterize the path after the feature writer creates my html to use in the shutdown script.
Reply
Enter your username or e-mail address. We'll send you an e-mail with instructions to reset your password.